We have various ways to achieve the result. However, we will discuss here two methods.
Table of Contents
1. Distinct
db.collection.distinct(field, query, options)
Finds the distinct values for a specified field across a single collection or view and returns the results in an array.
This method takes the following parameters:
Parameter | Type | Description |
---|---|---|
field | string | The field for which to return distinct values. |
query | document | A query that specifies the documents from which to retrieve the distinct values. |
options | document | Optional. A document that specifies the options. See Options. |
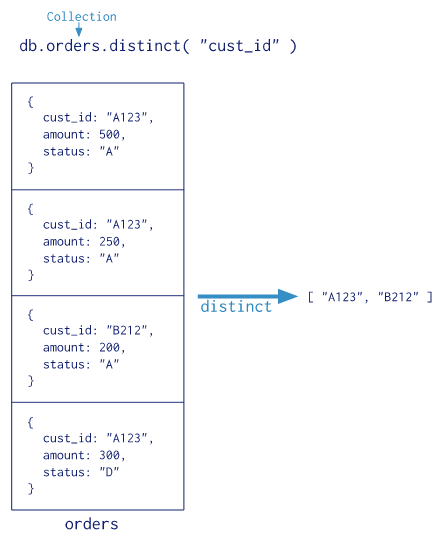
Examples
The examples use the inventory
collection that contains the following documents:
{ "_id": 1, "dept": "A", "item": { "sku": "111", "color": "red" }, "sizes": ["S", "M"] }{ "_id": 2, "dept": "A", "item": {"sku": "111","color": "blue" }, "sizes": [ "M", "L"] }{ "_id": 3, "dept": "B", "item": {"sku": "222", "color": "blue"}, "sizes": "S" }{ "_id": 4, "dept": "A", "item": {"sku": "333", "color": "black"}, "sizes": [ "S"] }
Return Distinct Values for a Field
The following example returns the distinct values for the field dept
from all documents in the inventory
collection:
db.inventory.distinct( "dept")
The method returns the following array of distinct dept
values:
[ "A", "B" ]
Return Distinct Values for an Embedded Field
The following example returns the distinct values for the field sku
, embedded in the item
field, from all documents in the inventory
collection:
db.inventory.distinct("item.sku")
The method returns the following array of distinct sku
values:
[ "111", "222", "333" ]
2. Aggregation Pipeline:
The following aggregation operation uses the $group
stage to retrieve the distinct item values from the sales
collection:
db.inventory .aggregate( [ { $group : { _id : "$dept" } } ] )
The operation returns the following result:
{ "_id" : "A" }{ "_id" : "B" }