Many times we want to draw a semi/half-circle or quarter circle or quarter circle with css in our design. It can be achieved with images, but for a few, it is difficult to create an image. It is easy to create any type of circle using CSS.
border-radius: 15px 50px 150px 150px; (first value applies to top-left corner, second value applies to top-right corner, third value applies to bottom-right corner, and fourth value applies to bottom-left corner):
border-radius
Table of Contents
1. Full-Circle:
First we will start with full circle
.full-circle { width: 300px; height: 300px; border-radius:50%; background-color: rgba(196, 196, 196, 1); }
Even full circle can be achieve like this also
.full-circle { width: 300px; height: 300px; border-radius:150px 150px 150px 150px; /* half of heght*/ background-color: rgba(196, 196, 196, 1); }
2. Semi-Circle:
If you want to create bottom or top semi-circle then your width is double of height.
1. Top semi circle
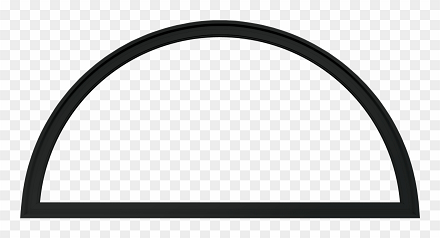
- To create top-semi-circle, height should be half of width.
- top-left corner and top-right corner of border-radius should be half of width.
- bottom-left corner and bottom-right corner of border-radius should be 0
.top-semi-circle { width: 300px; height: 150px; /* half of width*/ border-radius:150px 150px 0px 0px; background-color: rgba(196, 196, 196, 1); }svn unable to create pristine install stream
2. Bottom Semi Circle
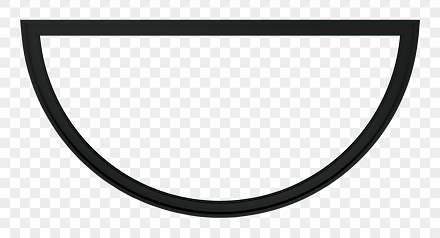
- To create bottom-semi-circle, height should be half of width.
- top-left corner and top-right corner of border-radius should be 0.
- bottom-left corner and bottom-right corner of border-radius should be half of width
.bottom-semi-circle { width: 300px; height: 150px; /*half of width*/ border-radius:0 0 150px 150px; background-color: rgba(196, 196, 196, 1); }
Now suppose you want to create left or right semi-circle in that case your height will be double of width.
3. Left Semi Circle
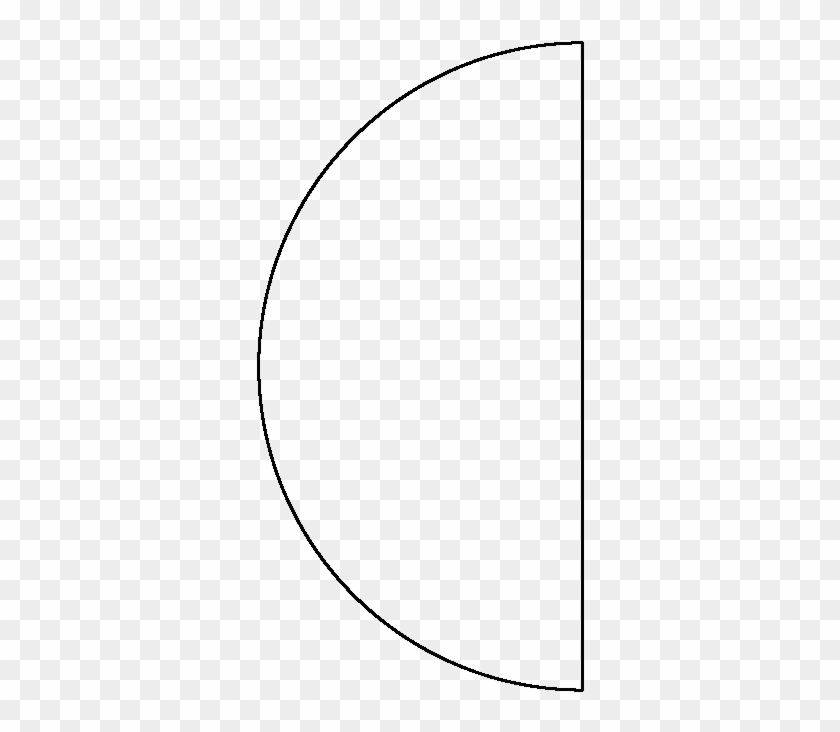
- To create left-semi-circle, height should be double of width.
- top-left corner and bottom-left corner of border-radius should be equal to width.
- top-right corner and bottom-right corner of border-radius should be 0.
.left-semi-circle { width: 150px; /* half of width*/ height: 300px; border-radius: 150px 0 0 150px; background-color: rgba(196, 196, 196, 1); }
Also Read : Get day, month and year Difference in JavaScript
4. Right Semi Circle
- To create left-semi-circle, height should be double of width.
- top-left corner and bottom-left corner of border-radius should be 0.
- top-right corner and bottom-right corner of border-radius should equal to width.
.right-semi-circle { width: 150px; /* half of width*/ height: 300px; border-radius: 0 150px 150px 0; background-color: rgba(196, 196, 196, 1); }
3. Quarter-Circle with CSS:
If we want to create a quarter circle in that case height and width should be equal:
1. Top Left Quarter Circle
- To create top left quarter circle, height should be equal to width.
- top-left corner of border-radius should be equal to width.
- top-right corner, bottom-right corner and bottom-left corner of border-radius should 0.
.top-left-quarter-circle { width: 300px; height: 300px; border-radius: 300px 0 0 0; background-color: rgba(196, 196, 196, 1); }
2. Top Right Quarter Circle
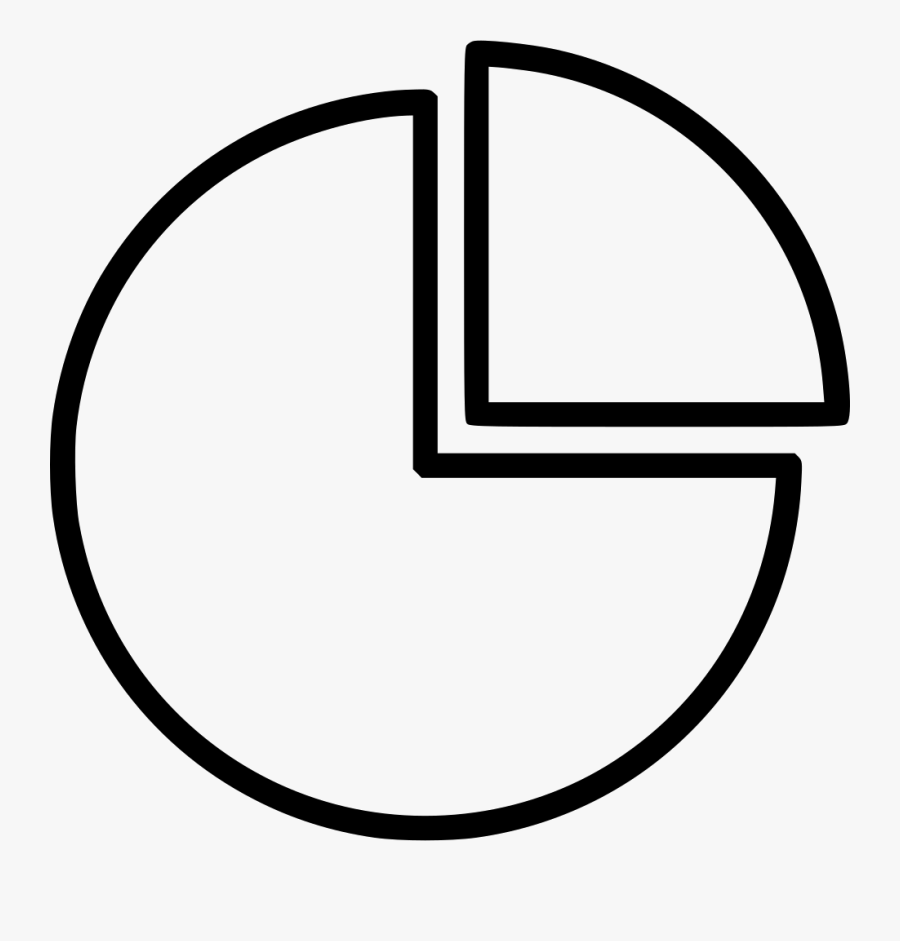
- To create top right quarter circle, height should be equal to width.
- top-right corner of border-radius should be equal to width.
- top-left corner, bottom-right corner and bottom-left corner of border-radius should 0.
.top-right-quarter-circle { width: 300px; height: 300px; border-radius: 0 300px 0 0; background-color: rgba(196, 196, 196, 1); }
Also Read: Angular: All about pipes template in angular
3. Bottom Right Quarter Circle
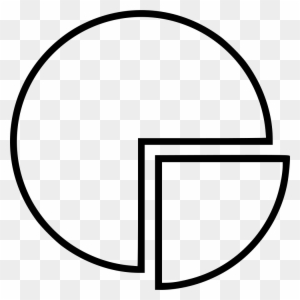
- To create bottom right quarter circle, height should be equal to width.
- bottom-right corner of border-radius should be equal to width.
- top-left corner, top-right corner and bottom-left corner of border-radius should 0.
.bottom-right-quarter-circle { width: 300px; height: 300px; border-radius: 0 0 300px 0px; background-color: rgba(196, 196, 196, 1); }
4. Bottom left Quarter Circle
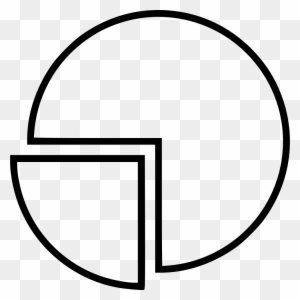
- To create bottom left quarter circle, height should be equal to width.
- bottom-left corner of border-radius should be equal to width.
- top-left corner, bottom-right corner and top-left corner of border-radius should 0.
.bottom-left-quarter-circle { width: 300px; height: 300px; border-radius: 0 0 0 300px; background-color: rgba(196, 196, 196, 1); }
Code: Create Circles using CSS
Reference : CSS Border-radius