We will see the example of $mul, $min, and $max in the update query of MongoDB. How we can use these update query field operators in our update query to get desirable results.
Table of Contents
1. Dummy user Data set :
[{name:'Max', age:34, isHealthy:true, salary:50}, {name:'Min', age:40, isHealthy:false, salary:100}, {name:'Mul', age:24, isHealthy:true}]
Insert data into db:
- Start Mongo DB. server.
- Now with Mongo DB server using command mongo
- Run command to create users DB. use users
- Now insert data into DB.
db.user.insertMany([{name:'Max', age:34, isHealthy:true, salary:50}, {name:'Min', age:40, isHealthy:false, salary:100}, {name:'Mul', age:24, isHealthy:true}])
- Now you have the required data to perform the operation.
db.user.count()
ordb.user.find({})
2. $min
$min
is helpful when we want to enforce any max criteria. If we want to set criteria that user age should not max than 25, if any age is above 25 then update age with 25.
syntax: { $min: { <field1>: <value1>, ... } }
If the field does not exist, the $min
operator sets the field to the specified value.
Statement : update all document with max age 25.
Query : db.user.updateMany({},{$min:{age:25}})
Result : { "acknowledged" : true, "matchedCount" : 3, "modifiedCount" : 2 }
Suppose now we want that max age should be 26 instead 25.
Query : db.user.updateMany({},{$min:{age:26}})
Result: { "acknowledged" : true, "matchedCount" : 3, "modifiedCount" : 0 }
As you can see modifiedCount
is 0 means nothing to update because all age is below 26 so, the update query will not update anything.
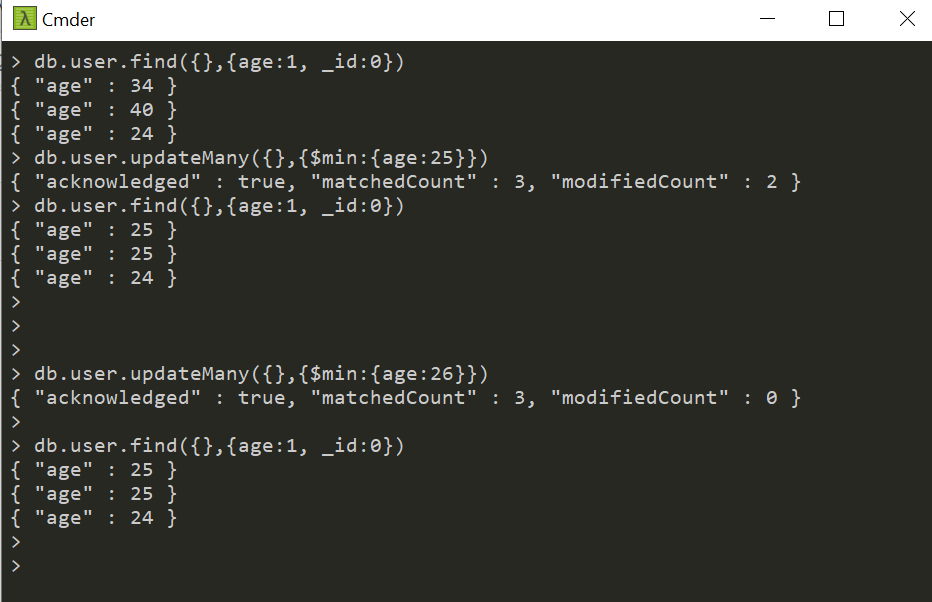
The
Mongo DB docs$min
updates the value of the field to a specified value if the specified value is less than the current value of the field. The$min
operator can compare values of different types, using the BSON comparison order.
3. $max
$max
is helpful when we want to enforce any min criteria. If we want to set criteria that user age should not be minimum than 28, if any age is below 28 then update age with 28.
syntax: { $max: { <field1>: <value1>, ... } }
If the field does not exists, the $max
operator sets the field to the specified value.
Statement : update all document with min age 28.
Query : db.user.updateMany({},{$max:{age:28}})
Result : { "acknowledged" : true, "matchedCount" : 3, "modifiedCount" : 3 }
Suppose now we want that min age should be 27 instead 28.
Query : db.user.updateMany({},{$max:{age:27}})
Result: { "acknowledged" : true, "matchedCount" : 3, "modifiedCount" : 0 }
As you can see modifiedCount
is 0 means nothing to update, because all age is above 27 so, the update query will not update any document.
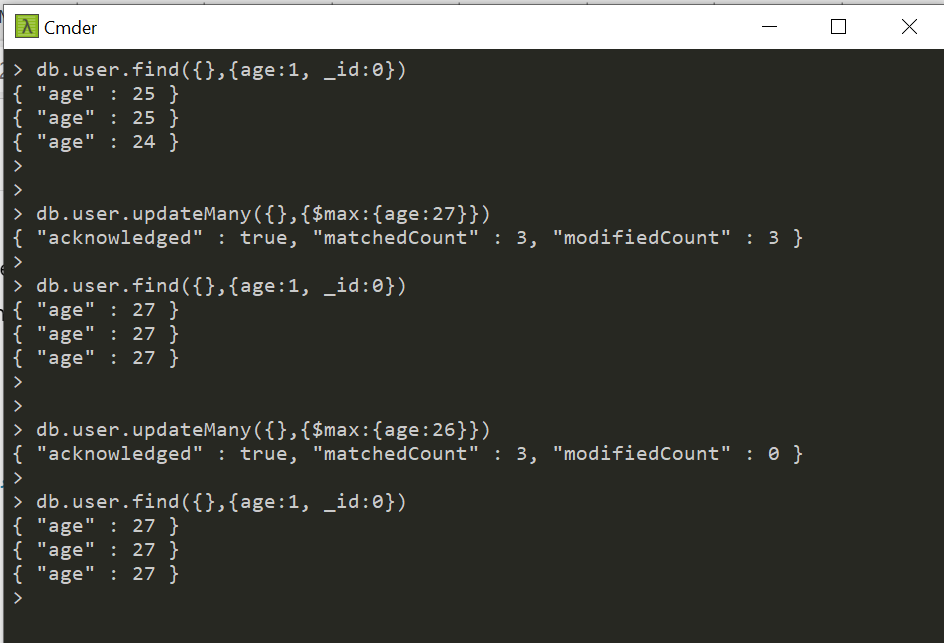
The
Mongo DDB doc$max
operator updates the value of the field to a specified value if the specified value is greater than the current value of the field. The$max
operator can compare values of different types, using the BSON comparison order.
4. $mul
$mul
is helpful when we want to increment or decrement any int value by some value. Suppose you want to increase the salary of every clerk by 10% or decrease the
salary of every board member by 5% then you can use $mul
operator.
syntax: { $mul: { <field1>: <number1>, ... } }
- The field to update must contain a numeric value.
- If the field does not exist in a document,
$mul
creates the field and sets the value to zero of the same numeric type as the multiplier.
Statement : update all document salary by 15%.
Query : db.user.updateMany({},{$mul:{salary:1.15}})
(100% + 15% = 1.15)
Result : { "acknowledged" : true, "matchedCount" : 3, "modifiedCount" : 3 }
Suppose now we want that decrease salary by 1%.
Query : db.user.updateMany({},{$mul:{salary:0.99}})
(100% – 1% = 0.99)
Result: { "acknowledged" : true, "matchedCount" : 3, "modifiedCount" : 3 }
Now we will see another example where we want to increase age by 1% and salary by 2%
Query: db.user.updateMany({},{$mul:{salary:1.02, age:1.01}})
Result: { "acknowledged" : true, "matchedCount" : 3, "modifiedCount" : 3 }
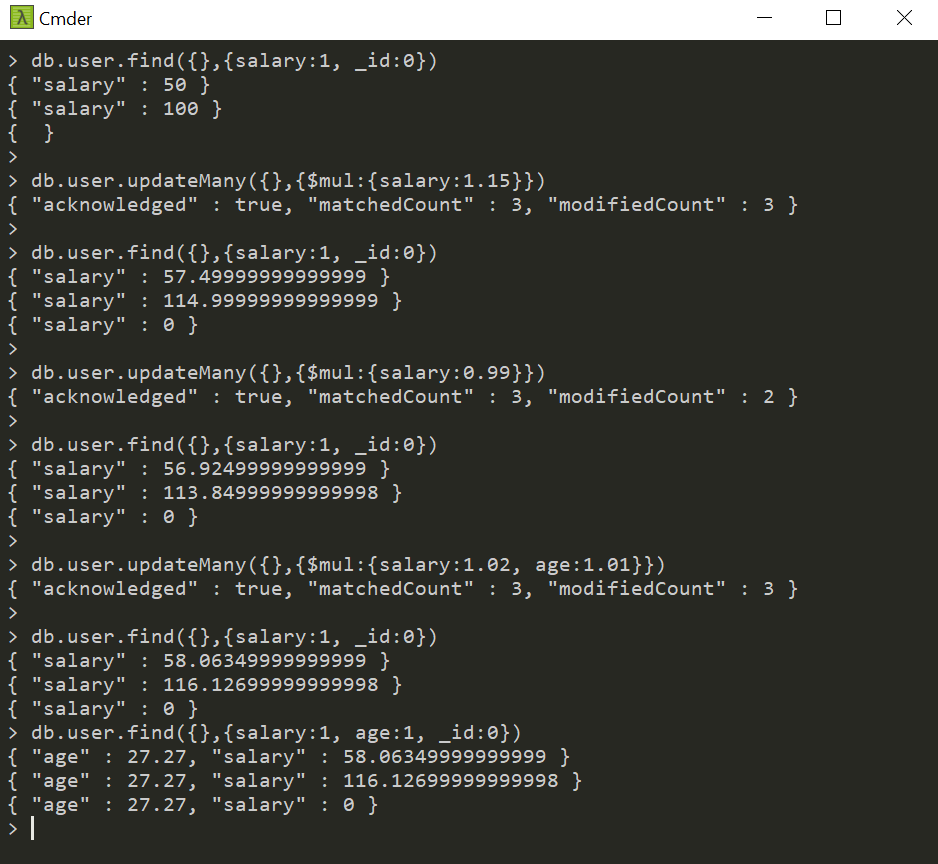