The insertMany()
is used to insert one or more document in the collection. It take an array of documents to insert into the collection. By default it insert document in the same order in which we gave in array of document.
Table of Contents
1. Syntax:
db.collection.insertMany( [ <document 1> , <document 2>, ... ], { writeConcern: <document>, ordered: <boolean> } )
Parameter | Type | Description |
---|---|---|
document | document | An array of documents to insert into the collection. |
writeConcern | document | Optional. To override existing write concerns we can use this write concern. Do not explicitly set the write concern for the operation if run in a transaction. |
ordered | boolean | Optional. It tells whether the mongod instance should perform an ordered or unordered insert. Defaults to true . |
2. Insert One document in collection
use flightDB db.flightDB.insertMany([ { "departureAirport": "MUC", "arrivalAirport": "SFO", "aircraft": "Airbus A380", "distance": 12000, "intercontinental": true }])
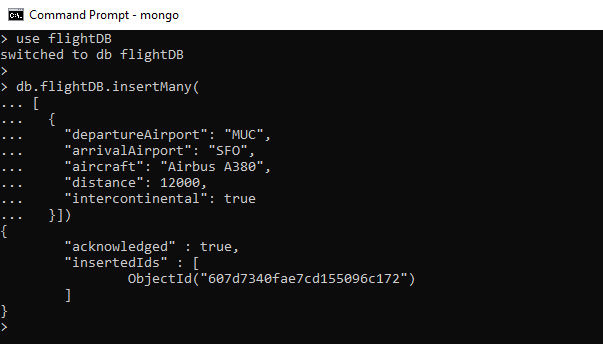
3. Insert more than one document
use flightDB db.flightDB.insertMany([ { "departureAirport": "LHR", "arrivalAirport": "TXL", "aircraft": "Airbus A320", "distance": 950, "intercontinental": false }, { "departureAirport": "MOC", "arrivalAirport": "SOO", "aircraft": "Airbus AB380", "distance": 22000, "intercontinental": true }])
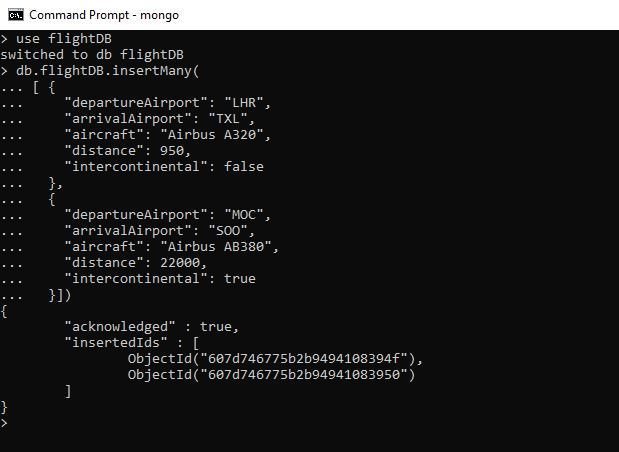
4. Insert document with custom Id
use flightDB db.flightDB.insertMany([ { "_id":"FA1", "departureAirport": "LHR", "arrivalAirport": "TXL", "aircraft": "Airbus A320", "distance": 950, "intercontinental": false },{ "_id":"FA2", "departureAirport": "MOC", "arrivalAirport": "SOO", "aircraft": "Airbus AB380", "distance": 22000, "intercontinental": true }])
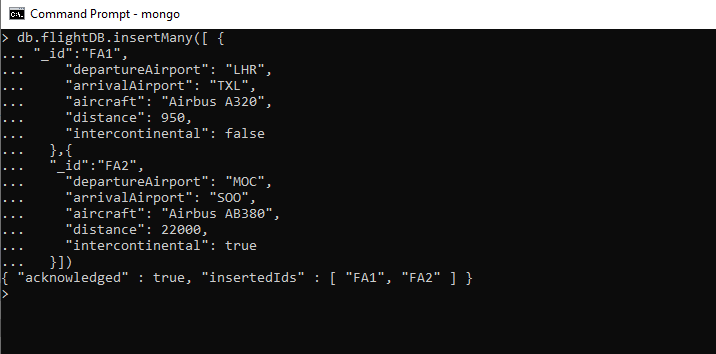
Now we will check how optional argument “ordered” affect our insertMany. To check this parameter we will insert some duplicate id elements.
5. Insert document with ordered parameter
1. Insert document with ordered:true
db.flightDB.insertMany([ { "_id":"FA1", "departureAirport": "LHR", "arrivalAirport": "TXL", "aircraft": "Airbus A320", "distance": 950, "intercontinental": false },{ "_id":"FA1", "departureAirport": "MOC", "arrivalAirport": "SOO", "aircraft": "Airbus AB380", "distance": 22000, "intercontinental": true },{ "_id":"FA3", "departureAirport": "LHRM", "arrivalAirport": "TMXL", "aircraft": "Airbus AM320", "distance": 1950, "intercontinental": false }], {ordered:true})
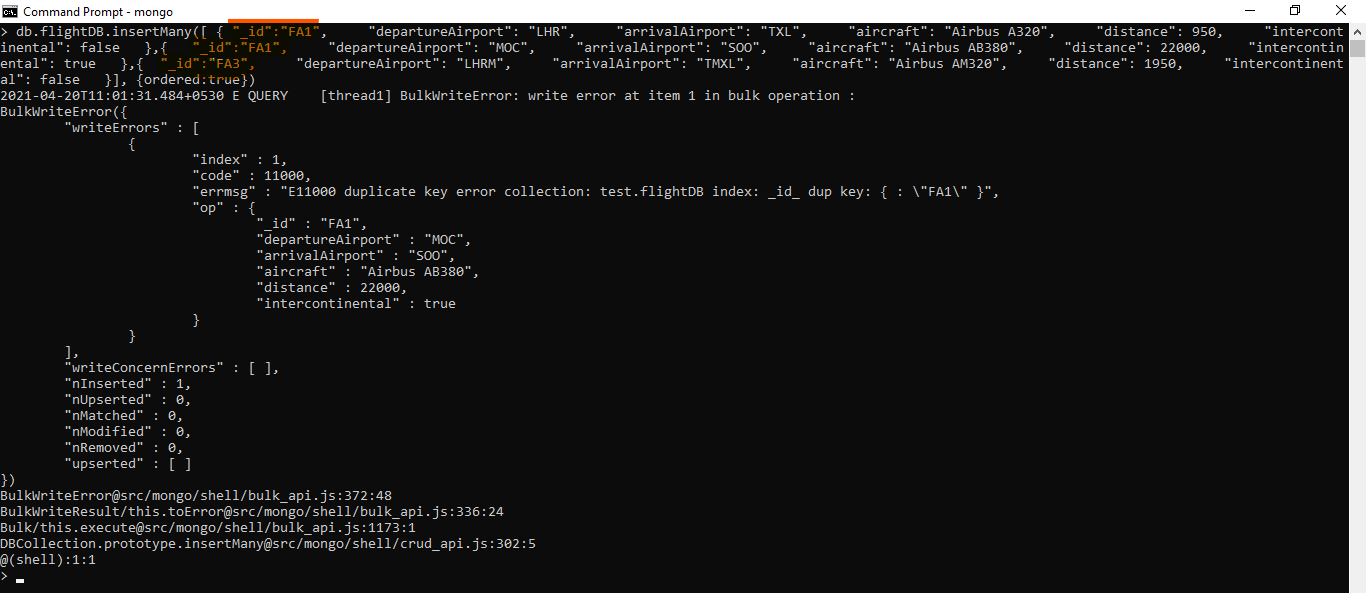
In the above code snippet, we can see we are trying to insert three documents and two documents have the same _id
. When we are trying to insert these document with optional parameter ordered:true
(by default it is true).
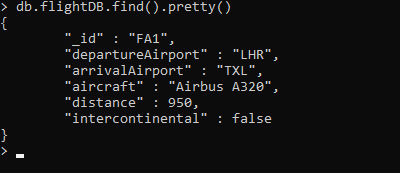
After insertion of first document it throw error and stop inserting other documents, it is due to ordered:true
. Now we will try with ordered:false
after removing all document.
2. Insert document with ordered:false
db.flightDB.insertMany([ { "_id":"FA1", "departureAirport": "LHR", "arrivalAirport": "TXL", "aircraft": "Airbus A320", "distance": 950, "intercontinental": false },{ "_id":"FA1", "departureAirport": "MOC", "arrivalAirport": "SOO", "aircraft": "Airbus AB380", "distance": 22000, "intercontinental": true },{ "_id":"FA3", "departureAirport": "LHRM", "arrivalAirport": "TMXL", "aircraft": "Airbus AM320", "distance": 1950, "intercontinental": false }], {ordered:false})
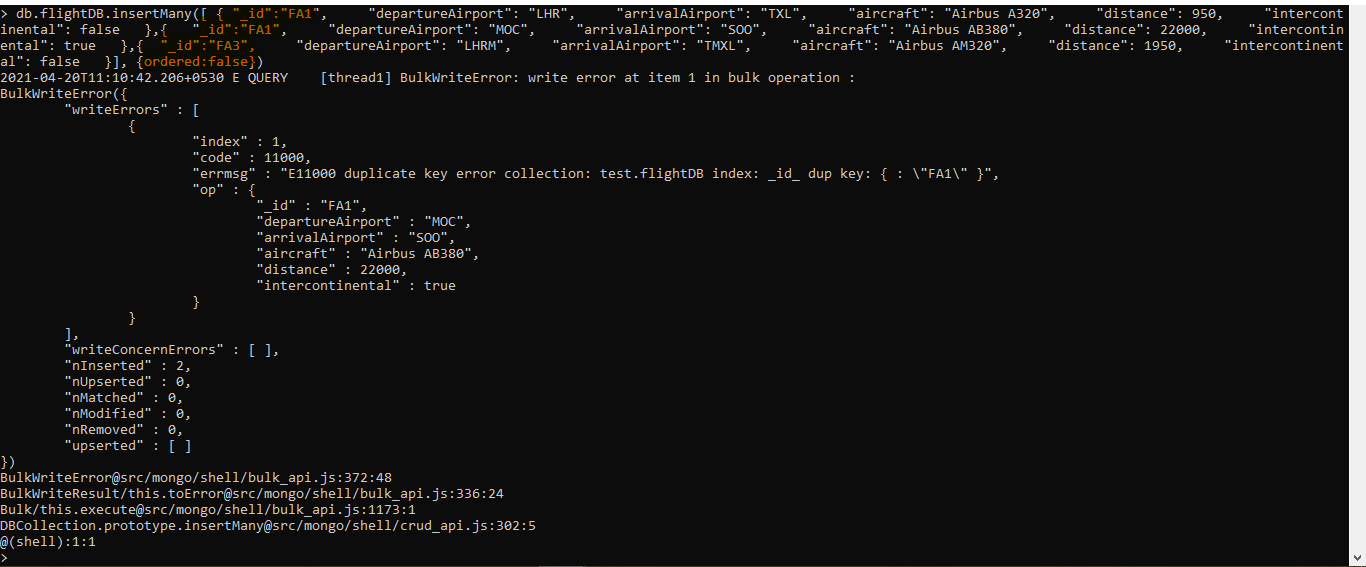
In the above code snippet, we can see we are trying to insert three documents and two documents have the same _id
. When we are trying to insert these document with optional parameter ordered:false
. Even after an error, it inserts all other documents. You can see nInserted:2
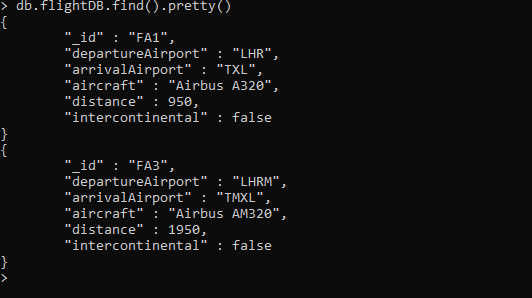
It is the benefits of ordered:false
, means if you do not care about failure but want to insert all document even after error then you can use ordered:false.
6. Insert document with writeConsern parameter
Write concern describes the level of acknowledgment requested from MongoDB for write operations to a standalone mongod
or to replica sets or to sharded clusters.
- the
w
option to request acknowledgment that the write operation has propagated to a specified number ofmongod
instances or tomongod
instances with specified tags. - the j option to request acknowledgment that the write operation has been written to the on-disk journal, and
- the wtimeout option to specify a time limit to prevent write operations from blocking indefinitely.
{ w: <value>, j: <boolean>, wtimeout: <number> }
1. with w:1
Requests acknowledgment that the write operation has propagated to the standalone mongod
or the primary in a replica set. w: 1
is the default write concern for MongoDB.
If we want any acknowledgment after insert operation then we use w:1
(by default it is one)
db.flightDB.insertMany([ { "_id":"FA2", "departureAirport": "LHR", "arrivalAirport": "TXL", "aircraft": "Airbus A320", "distance": 950, "intercontinental": false },{ "_id":"FA1", "departureAirport": "MOC", "arrivalAirport": "SOO", "aircraft": "Airbus AB380", "distance": 22000, "intercontinental": true },{ "_id":"FA3", "departureAirport": "LHRM", "arrivalAirport": "TMXL", "aircraft": "Airbus AM320", "distance": 1950, "intercontinental": false }], {w:1})

2. with w:0
Requests no acknowledgment of the write operation. However, w: 0
may return information about socket exceptions and networking errors to the application. Means we do not want any acknowledge after insertion.
db.flightDB.insertMany([ { "_id":"FA2", "departureAirport": "LHR", "arrivalAirport": "TXL", "aircraft": "Airbus A320", "distance": 950, "intercontinental": false },{ "_id":"FA1", "departureAirport": "MOC", "arrivalAirport": "SOO", "aircraft": "Airbus AB380", "distance": 22000, "intercontinental": true },{ "_id":"FA3", "departureAirport": "LHRM", "arrivalAirport": "TMXL", "aircraft": "Airbus AM320", "distance": 1950, "intercontinental": false }], {w:0})

As you can see in above pic there is no acknowledge of insertion as you receive when w:1
was there.