Here are my python interview/job preparation questions and answers. Which can help to to clear the interview or also help to take interview of any candidates.
Table of Contents
What is list ?
List can be written as a list of comma-separated values (items) between square brackets. Lists might contain items of different types, but usually the items all have the same type.
Lists are a mutable type, i.e. it is possible to change their content.
squares = [1, 4, 9, 16, 25]
nameList = ['p','y','t','h','o','n']
What is difference between append, extend and insert ?
append : append
object to the end of the list.
append(object)
squares = [1,4,9,16]
squares.append(25) # [1,4,9,16,25]
squares.append([36,49]) # [1,4,9,16,25, [36,49]]
If we append a list in a list then it will not expand the list instead append complete list
insert : inserts
an element to the list at the specified index.
insert(index, object)
squares = [1,4,9,16]
squares.insert(1,25) # [1,25,4,9,16]
squares.insert(10,[36,49]) # [1,4,9,16,25, [36,49]]
If we give index position which is out of length then it will insert at end.
extend : Extend list by appending elements from the iterable.
squares = [1,4,9,16]
squares.extend([36,49]) # [1,4,9,16,6,49]
squares.extend(64) # [1,4,9,16,6,49,64]
What is the difference between remove, del and pop?
remove : Remove first occurrence of value. Raises ValueError if the value is not present.
remove(value)
squares = [1,4,9,16]
squares.remove(4)
squares # [1,9,16]
pop: Remove and return item at index (default last).
pop(index=-1)
squares = [1,4,9,16]
squares.pop() #16
squares.pop(2) # 9
del : removes an element by index.
squares = [1,4,9,16]
del squares[0]
del #[1,4,9,16]
How do we interchange the values ?
a = 10, b = 12
b,a = a,b
print(a,b) #12,10
c = [2,3], d = [1,2]
d,c = c,d
print(c,d) # [1,2], [2,3]
What is lambda in Python?
In Python, an anonymous function is a function that is defined without a name. Anonymous functions are also called lambda functions.
lambda arguments: expression
# Program to show the use of lambda functions
square= lambda x: x * x
print(square(15)) # 225
Also Read: Python – Basic Data Types
What is different way copy a list ?
We can copy a list three ways, one is normal way, shallow copy and other is deepcopy.
- In normal way copy i.e. reference copy we actually copy the reference not only value.
l1 = [1,2,3]
l2 = l1
l1.append(4)
print(l1) #[1,2,3,4]
print(l2) #[1,2,3,4]
- In shallow copy if list is normal list i.e. not a nested list then shallow copy is useful. There is any change in original list does not affect copied list and vice versa. But if it is nested list then any change in nested list then changes will reflect in both original as well as copied list.
- For shallow copy you can use one of the following method
list(list_name_to_copy)
<list_name_to_copy>.copy()
<list_name_to_copy>[:]
l1 = [1,2,3]
l2 = list(l1)
l1.append(4)
print(l1) #[1,2,3,4]
print(l2) #[1,2,3]
li3 = [['a'],['b'],['c']]
li4 = list(li3) // OR you can use li3.copy() OR li3[:] (list comprehension)
li3.append([4])
print(li4)#[['a'],['b'],['c']]
print(li3) #[['a'],['b'],['c'], [4]]
#if we change in nested element then li3 and li4 will reflect the changes
li3[0].append('e')
print(li4)#[['a','e'],['b'],['c']]
print(li3) #[['a','e'],['b'],['c'], [4]]
- In deep copy if we change in nested list of list of original list than it will not change copied list and vice versa
- for deep copy you need to
import copy
and usecopy.deepcopy()
- for deep copy you need to
import copy
li3 = [['a'],['b'],['c']]
li4 = copy.deepcopy(li3)
li3.append([4])
li3[0].append('e)
print(li4)#[['a'],['b'],['c']]
print(li3) #[['a', 'e'],['b'],['c'], [4]]
What is the difference between a list and a tuple?
lists and tuples are data structures in Python. Both are sequential data structure. In both element can be access with the help of index. Both can store homogenous as well as heterogenous data elements.
List | Tuple |
---|---|
Lists are mutable | Tuples are immutable |
Methods that add items or remove items are available with list. | Methods that add items or remove items are not available with tuple. |
We can delete or remove items from a list | We cannot delete or remove items from a tuple. |
List elements cannot be used as a key for a dictionary. | Tuples that contain immutable elements can be used as a key for a dictionary |
Iterations is Time-consuming | Iterations is less Time-consuming than list |
Also Read : Python: Operators and Expressions
What is the difference between “is” and “==”?
Sometime it feel both work similar way but there is a catch. But both work different way to check equality.
- Use
is
to check identity and==
to check equality. ==
compares the values of the objects.is
compares the references of the objects.==
is for value equality. Use it when you would like to know if two objects have the same value.is
is for reference equality. Use it when you would like to know if two references refer to the same object
How slice operator work with list
list[start:stop:step]
start | Optional. An integer number specifying at which position to start the slicing. Default is 0 |
end | An integer number specifying at which position to end the slicing |
step | Optional. An integer number specifying the step of the slicing. Default is 1 |
Some use cases of slice operator:
- Reverse the list
a = [1,2,3,4]
reverse_a = a[::-1] #[4,3,2,1]
name = "singhak"
print(name[::-1])// "kahgnis"
- Copy the list
a = [1,2,3,4]
b = a[:]
- Create sub list
a = [1,2,3,4,5,6]
print(a[2:]) #[3, 4, 5, 6]
print(a[2:4]) #[3, 4]
print(a[::2]) #
[1, 3, 5]
print(a[1:5:3]) #[2, 5]
What is negative index in Python?
With the help of negative index we can retrieve the element from list. In negative indexing -1 indicate last element of list and similarly -2 indicate second last element and so on.
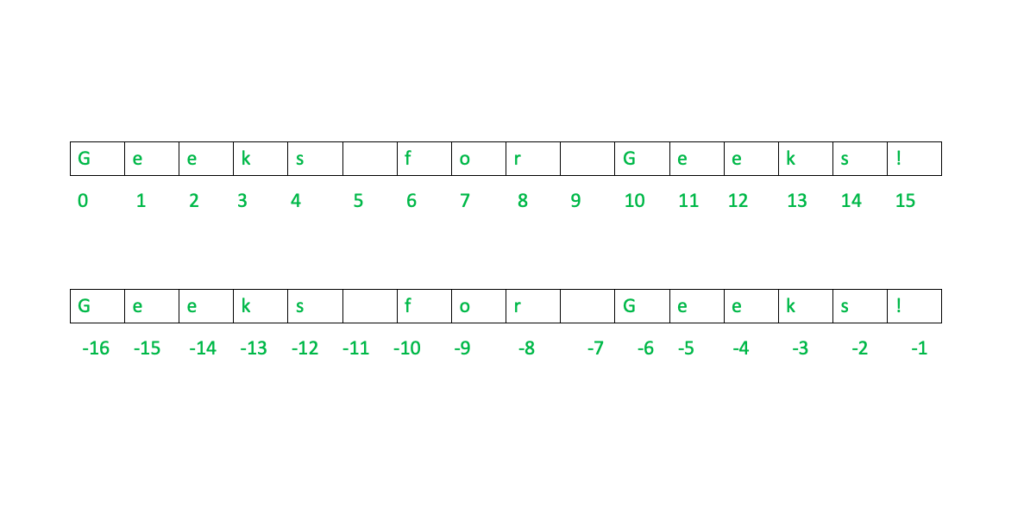