In ECMAScript 2020, also known as ES11 Nullish Coalescing operator introduce.
The nullish coalescing operator (??) is a logical operator that returns its right-hand side operand when its left-hand side operand is null or undefined and otherwise returns its left-hand side operand.
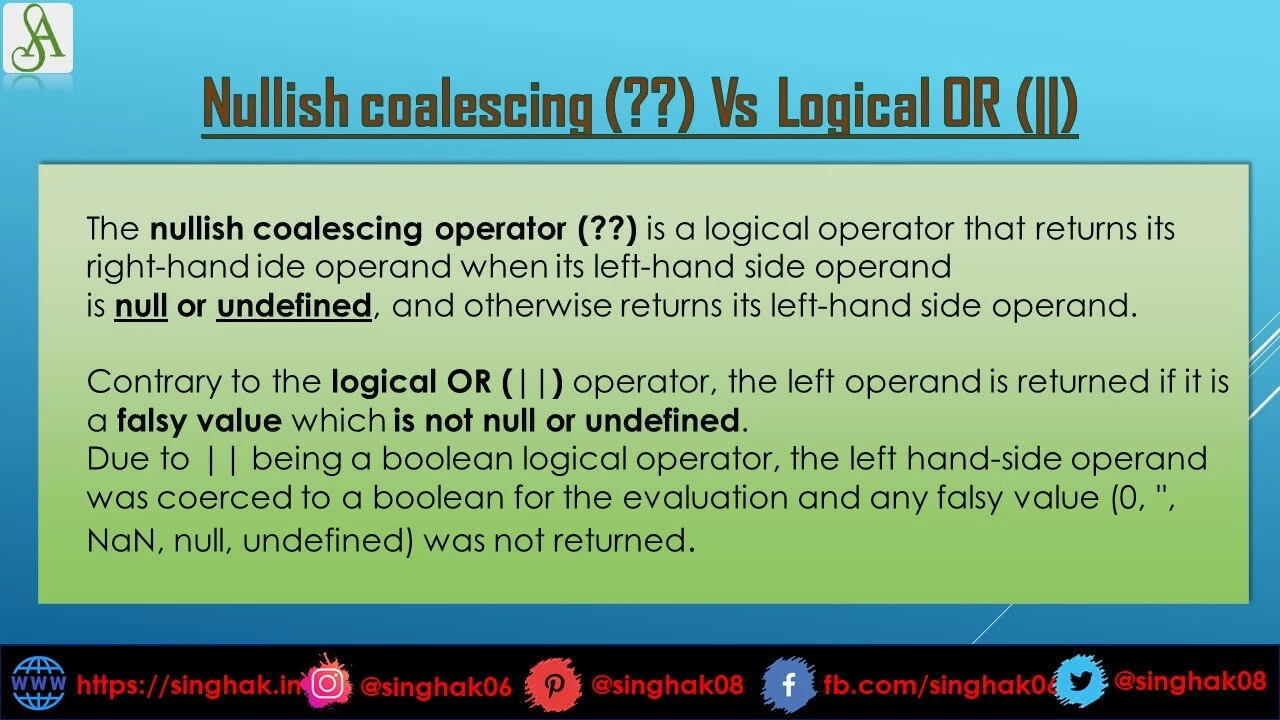
Assigning a default value to a variable
Earlier, we used a logical OR operator (||) to assign a default value to a variable.
let foo; // foo is never assigned any value so it is still undefined let someDummyText = foo || 'Hello!';
However, It works most of the time but in a few cases, it does not work as per requirement. This may cause unexpected consequences if you consider 0
, ''
, or NaN
as valid values.
CONTRARY TO the logical OR (||) operator, the left operand is returned if it is a falsy value which is not null or undefined
let num = 0 // suppose we want to assign num value myNumber let myNum = num || 1 console.log(myNum) // output : 1
As you observed in the above case we want to assign variable “num” as a default value to a new variable “myNum”. But it did not work since 0 is a falsy value, and even though it is a number, the “myNumber” variable will have the right-hand value assigned to it.
To solve this issue we have a new operator named Nullish coalescing operator. The nullish coalescing operator avoids this pitfall by only returning the second operand when the first one evaluates to either null
or undefined
(but no other falsy values).
leftExpr ?? rightExpr
let num = 0 // for logical operator 0 is falsy value // suppose we want to assign num value myNumber let myNum = num ?? 1 console.log(myNum) // output : 0
let myText = ''; // An empty string (which is also a falsy value) let notFalsyText = myText || 'Hello world'; console.log(notFalsyText); // Hello world let preservingFalsy = myText ?? 'Hi neighborhood'; console.log(preservingFalsy); // '' (as myText is neither undefined nor null)
Short-circuiting:
Like the OR and AND logical operators, the right-hand side expression is not evaluated if the left-hand side proves to be neither null nor undefined.
function A() { console.log('A was called'); return undefined;} function B() { console.log('B was called'); return false;} function C() { console.log('C was called'); return "foo";} console.log( A() ?? C() ); // logs "A was called" then "C was called" and then "foo" // as A() returned undefined so both expressions are evaluated console.log( B() ?? C() ); // logs "B was called" then "false" // as B() returned false (and not null or undefined), the right // hand side expression was not evaluated
No chaining with AND or OR operators:
It is not possible to combine both the AND (&&) and OR operators (||) directly with ??. A SyntaxError
will be thrown in such cases.
null || undefined ?? "foo"; // raises a SyntaxError true || undefined ?? "foo"; // raises a SyntaxError
However, providing parenthesis to explicitly indicate precedence is correct:
(null || undefined) ?? "foo"; // returns "foo"