While working on frontend most of the time we use console.log(). In sort for frontend developers, it is a tool for debugging. But most of the developer doesn’t know the real power of the console. It consists of lots of features a few of them I will discuss here.
Table of Contents
#1: Organized logging:
var chair = { type1:"Wooden", type2: "Iron", type3: "Plastic"} var table = { type1:"Wooden", type2: "Iron", type3: "Plastic"}
Normal Way logging:
console.log(chair, table)

Organized way logging, In this way we can easily identify the log variable.
console.log({chair, table})
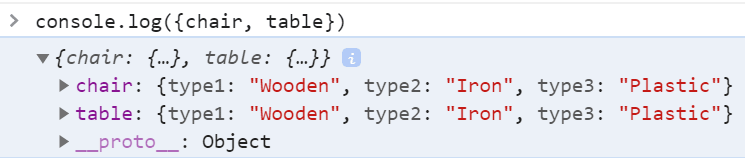
#2: Measure performance of code with the console.time():
Many times we want to measure the time taken by any piece of code to check the performance of code. There may be many ways to do but the easiest way is using the console methods.
Here is a trivial way to do.
const start = Date.now(); // do some stuff console.log('Took ' + (Date.now() - start) + ' millis');
An elegant way to achieve something similar
console.time('Label 1'); // do some stuff console.timeEnd('Label 1');
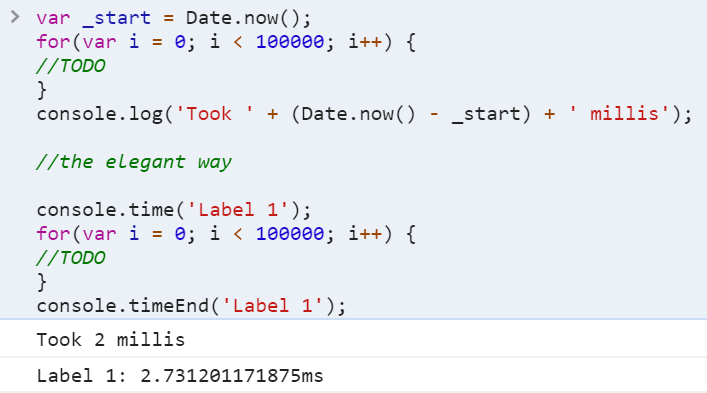
#3: Table – console.table()
Sometimes it’s a mess to see the structure of the objects printed by console.log()
. console provides a nice way of outputting objects to the console via the console.table()
method. It takes two arguments— data
and columns
, which is optional.
var x = [{name:'anil',age:23},{name:"akash",age:28}, {name:'anita',age:22},{name:"akashi",age:21}]
case 1: using normal console.log()
. console.log(x)
case 2: Using console.table()
method.console.table(x)
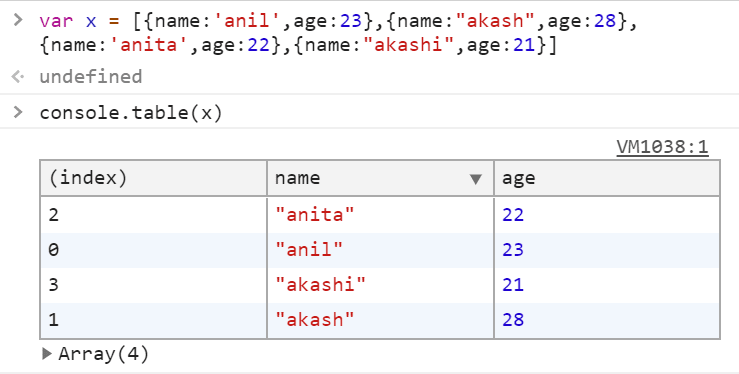
Even you can sort the data by clicking column name in the table
sorting the data
#4: Using groups in the console
Many times we want to group our logs for better debugging. Grouping the related logs make our life easy.
For this purpose, you can use nested groups to help organize your output by visually associating related messages. To create a new nested block, call console.group(). To exit the current group, call console.groupEnd(). For example, given this code:
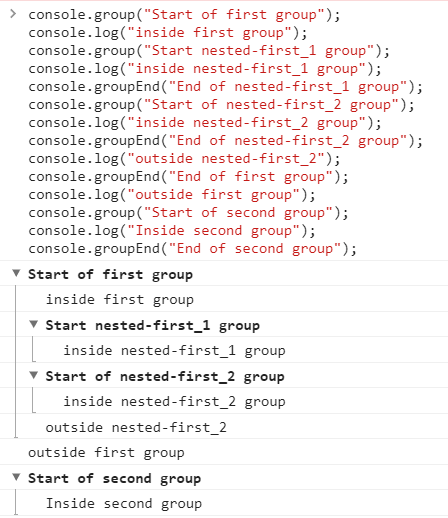
#5: Console.log, Console.error, Console.warn and Console.info
These are probably the most used methods of all. You can pass more than one parameter to these methods. Each parameter is evaluated and concatenated in a string delimited by the space, but in case of objects or arrays, you can navigate between their properties.
#6 console.trace() and console.assert():
The console.trace() the method displays a trace that shows how the code ended up at a certain point.
function foo() { function bar() { console.trace(); } bar(); } foo();
In the console, the following trace will be displayed:
console.trace bar @ VM2461:3 foo @ VM2461:5 (anonymous) @ VM2461:7
The console.assert() the method writes a message to the console, but only if an expression evaluates to false.
Calling console.assert() with a falsy assertion print message to the console without interrupting execution of subsequent code.
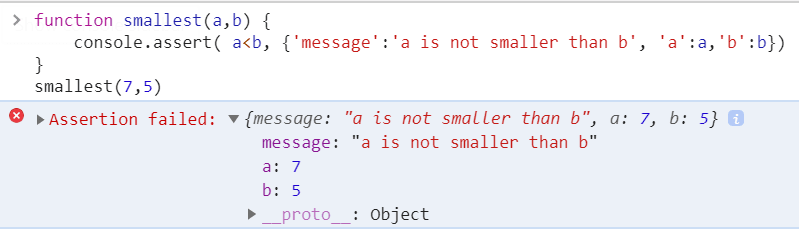
We will describe console.trace() and console.assert() in details in another blog. Along with these two we will tell few other intesting console tricks
will discuss in new blog
Read More: Map Vs forEach in javascript
References:
https://console.spec.whatwg.org/
https://developer.mozilla.org/en-US/docs/Web/API/Console